Adding a Rake Task for SQL Views to a Rails Project
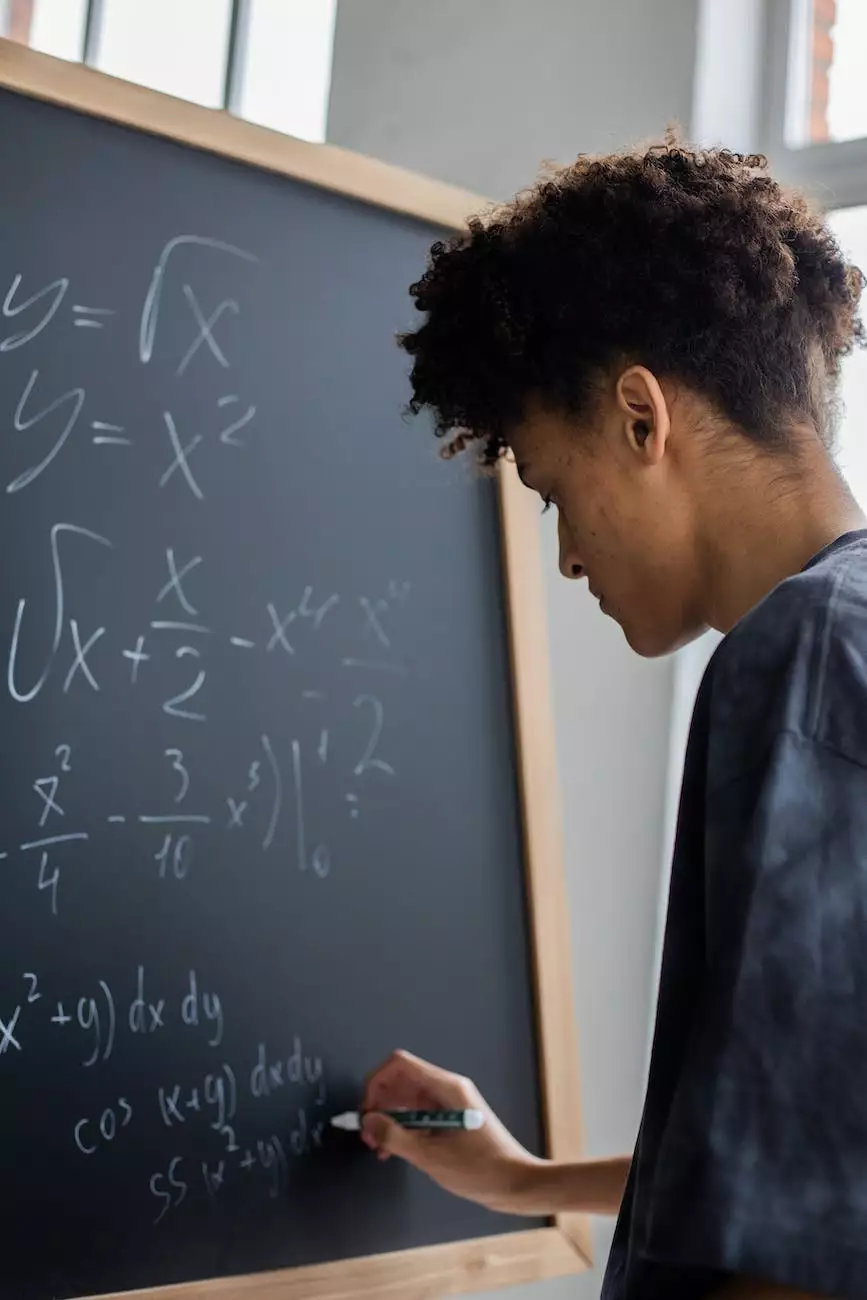
Introduction
Are you looking to leverage the power of SQL views in your Rails project? In this comprehensive guide brought to you by ATM Marketing Solutions, a leading website development company specializing in business and consumer services, we'll walk you through the process of adding a Rake task for SQL views to your Rails project. By implementing this powerful technique, you'll be able to optimize your database queries and improve the overall performance of your application.
Why Use SQL Views in Rails?
Before we dive into the technical details, let's explore why using SQL views can be beneficial for your Rails project. SQL views provide a convenient way to abstract complex database queries into manageable entities. By creating virtual tables with predefined queries, you can simplify your code and improve maintainability. Additionally, SQL views can significantly enhance performance by executing pre-optimized queries directly on the database level.
Step-by-Step Guide
Step 1: Generate a New Rake Task
The first step in adding a Rake task for SQL views is to generate a new Rake task file. Open your terminal and navigate to the root directory of your Rails project. Run the following command:
rails generate task sql_viewsThis command will generate a new file named sql_views.rake in the lib/tasks directory of your project.
Step 2: Implement the Rake Task
Open the sql_views.rake file in your preferred text editor. Inside the file, you'll see a generated skeleton for your Rake task. Replace the existing code with the following:
namespace :sql_views do desc 'Refresh SQL views' task :refresh => :environment do # Code to refresh SQL views end endIn the above code snippet, we define a new Rake namespace called :sql_views. Within this namespace, we create a task called :refresh. This task will be responsible for refreshing our SQL views. Feel free to modify this task to meet your specific requirements, such as using different naming conventions or incorporating additional logic.
Step 3: Writing the SQL View Refresh Logic
Now it's time to implement the logic to refresh our SQL views. In Rails, you can execute raw SQL queries using the ActiveRecord::Base.connection.execute method. Within the :refresh task, add the following code:
task :refresh => :environment do # Fetch the names of all SQL views in your project view_names = ActiveRecord::Base.connection.execute(" SELECT table_name FROM information_schema.tables WHERE table_schema = 'public' -- Replace 'public' with your schema name AND table_type = 'VIEW' ") # Loop through the view_names and refresh each view view_names.each do |view_name| ActiveRecord::Base.connection.execute("REFRESH MATERIALIZED VIEW #{view_name['table_name']}") end endThe code above retrieves the names of all SQL views in your project's schema using a raw SQL query. It then iterates through each view name and refreshes the corresponding materialized view using the REFRESH MATERIALIZED VIEW statement. Replace the 'public' schema name if you are using a different schema in your project.
Step 4: Running the Rake Task
Once you've implemented the SQL view refresh logic, you can easily execute the Rake task. Open a terminal, navigate to your Rails project's root directory, and run the following command:
rails sql_views:refreshUpon running the command, Rails will execute your custom Rake task, refreshing all SQL views defined in your project. You can incorporate this task into your deployment process or run it manually whenever you need to update your views.
Conclusion
Congratulations! You've successfully learned how to add a Rake task for SQL views to your Rails project. By leveraging the power of SQL views, you can optimize your database queries, improve performance, and simplify code maintenance. Implementing this technique will undoubtedly help you build high-quality, efficient web applications. Remember, if you need professional assistance with your website development needs, don't hesitate to reach out to ATM Marketing Solutions. Our team of experts is always ready to help you achieve your goals.